PYTHON: Turtle Graphics 
- Drawing a Star
- the user choosing the number of points
It wasn't until I started this project that I realised just how complex getting the computer to draw stars really is.... this is my analysis of the problem.
There are lots of ways of tackling it - but here is my solution....

Stars with an odd number of points
Stars with odd numbers of points proved to be easy (as I had already drawn a pentacle for Halloween!), but even number stars proved more of a challenge.
Stars with an even number of points
If you halve the number of points on stars that have a even total of points - you get two classes of star - those that yield an odd number of points when halved and those when halved yield an even number of points.
Stars with and even number of points, that halves to an odd number (like 6, 10, 14...)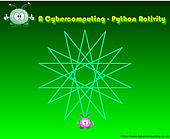
You can draw a star with and even number of points, that halves to an odd number (like 6, 10, 14...) by constructing the odd number half star - then producing an inverted version of it on top.
Stars with and even number of points, that halves to an even number (like 8, 12, 16...)
To draw these stars I constructed overlapping kite figures - rotating the kite each time before redrawing.
I then turned that into a flower drawing program,
- take a look!

First we have to import the libraries of commands we use in the program....
We are drawing figures, and also have to use mathematical expressions so we need the turtle and math libraries:
import turtle
import math
We then need to set up the screen:
screen=turtle.Screen()
screen.setup(550,450)
screen.bgpic("background.png")# sets the screen background to an image I have uploaded
screen.addshape("FuzzlePINK.png")# adds and image to the screen
Now, to define the 'turtle' - set a graphic as the cursor and decide on the colour and thickness of the line to be drawn...
fuzzle = turtle.Turtle("FuzzlePINK.png")# sets the turtle to a fuzzle graphic
fuzzle.shape("FuzzlePINK.png")# sets the shape to that of the graphic
fuzzle.color("mediumspringgreen")# there are lots of colours for you to use in the turtle mode of python!
fuzzle.width(2)#set width of drawn line
fuzzle.up()#lifts the pen up so we can move the Fuzzle from the centre of the screen to our chosen starting point.
fuzzle.goto(0,130)#set position of the turtle at the top of the screen under the heading - but on the centreline of screen
fuzzle.down()#puts the pen back down, so when we move it - it will draw a line.
Now to set our variables to zero before we get started and to put in some calculations for our figures.
It is best to put these together so you can easily find them if you wish to change something later - or add to them:
count=0
number=0
side=200
height=math.sqrt(0.75*side*side)
numbertype="unknown"
Now the information to be given to the user.
This is important - information and instructions are vital so that best use is made of your program.
print("This program aims to draw stars with any number of points that you request - but it is not able to draw a star with less that four points... and stars with more that 125 points take ages and have lots of overlap - so I wouldn't request those if I was you!")
print("")
print("")#spacing out of info is necessary to make it easy to use.
Now for the main program!
We need an input - but it has to be an integer and between parameters that make the drawing of the figure possible. We can therefore use 'while True' to ask for an integer - if anything else is entered there will be a 'ValueError' and that can give a response and get the user to try again...
while True:
try:
number=int(input("Please choose the number of points you want the star to have:"))
break
except ValueError:
print("")
print("")
print("You can only enter whole numbers - not decimals, letters or symbols.")
print("")
Now we have an integer entered by the user we have to find out whether it lies within the parameters we have decided will 'work' for the program:
while number<5 or number>125:#Guiding the user to choose a number in a range that will be sensible
if number<4:
print("")
print("")
print("To draw a star you must have a minimum of 4 points...")
if number>125:
print("The number of points you have chosen is so great that the points would be too close together and the figure would look like a circle.")
print("")
print("")
number=int(input("Please input the number of sides you want on the figure:"))
Now we have an integer within the correct range we now have to sort the figures out into three sets: odd numbers, even numbers that are a double of an odd number and even numbers that are a double of an even number.
else:#Finding out if the number is odd or even
if(number % 2)==0:#Checking to see if divided by two there is a remainder
numbertype="even"
Now - I discovered that I could draw even number star points that halve to an odd number by using my 'odd' points program instruction - duplicated when inverted over... but I had to use multiple kite shapes for the other even number ones (the ones that when halved twice still give an even number as the answer).
evennumber=number/2#Checking to see if when divided by two there is a remainder
if(evennumber % 2)==0:
eventype="doubleEven"
fuzzle.up()
fuzzle.goto(0,-35)#set position of the turtle at centre of screen
fuzzle.left(90)#set turtle pointing up
count=0
fuzzle.down()
while count<number:
fuzzle.right(45)#This draws the kite
fuzzle.forward(54)
fuzzle.left(40)
fuzzle.forward(150)
fuzzle.left(160)
fuzzle.forward(150)
fuzzle.left(40)
fuzzle.forward(54)
fuzzle.right(195)
count=count+1
fuzzle.left(360/number)
else: count=0
fuzzle.up()
quit()#This stops the program after drawing the figure
else:
eventype="singleEven"
fuzzle.rt(90)
while count<(number/2):#this draws the half of the star
fuzzle.right(180/(number))
fuzzle.forward(side)
fuzzle.left(180-(180/(number)))
count=count+1
else:
count=0
fuzzle.up()
fuzzle.goto(0,(130-(height+(height/(number/2)))))
fuzzle.rt(180)
fuzzle.down()
while count<(number/2):#this sraws the other half of the start (the inverted bit)
fuzzle.left(180/(number))
fuzzle.forward(side)
fuzzle.right(180-(180/(number)))
count=count+1
else:
count=0
else:
numbertype="odd"#Here the odd number of points figures are drawn
fuzzle.left(90-(90/(number)))
while count<number:
fuzzle.rt(180-(360/(2*number)))
fuzzle.forward(side)
count=count+1
else:count=0
fuzzle.seth(90)#finishes with the Fuzzle upright