PYTHON: A Turtle Graphics Project
A Halloween Greeting 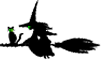
A Carved Pumpkin (October 2022)
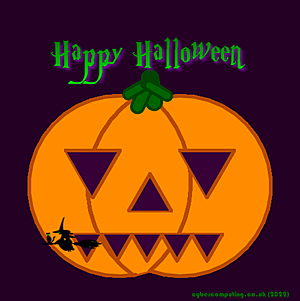
This is a python program that draws a Halloween Pumpkin Greeting:
We start off by importing the turtle module into our program:
import turtle
#Set variables to zero
count=0
We now have to set up the screen.
I have decided to set the background for this program to a deep purple - the hex value of this I ascertained from a graphics program.
I have set the cursor to be a witch on a bloom (with her cat), and included a greeting graphic and a logo for my site.
These images have to be imported from my Trinket library - it takes png and gif images.
You can see how I incorporate the graphics below:
screen=turtle.Screen()
screen.setup(500,500)
screen.bgcolor("#240024")
screen.addshape("witchOnBroom60right2.png")
screen.addshape("HappyHalloween.png")
screen.addshape("logo.png")
I now need to set up the 'turtles'. The greeting image and the logo are 'turtles' - but the main one is the one that will have the witch as the graphic.
logo=turtle.Turtle("logo.png")#The 'logo' Turtle
logo.shape("logo.png")
logo.up()#It is necassary to lift up the turtle to move it without making a line on the screen
logo.rt(270)
logo.goto(150,-240)
logo.down()#Now it is where I want it - I can put it down!

text=turtle.Turtle("HappyHalloween.png")#The text Turtle
text.shape("HappyHalloween.png")
text.up()#It is necassary to lift up the turtle to move it without making a line on the screen
text.goto(0,150)
text.down()#Now it is where I want it - I can put it down!
text.rt(630)#Wheeling it around!
text.up()

turtle = turtle.Turtle("witchOnBroom60right2.png")#Set up turtle to draw pumpkin
turtle.shape("witchOnBroom60right2.png")#A witch will 'draw' the figure
turtle.color("#B74900")#The colour of the line drawn will be a very dark orange (hex colour selected from a graphics program)
turtle.fillcolor("darkorange")#The fill is selected from the named colours - see here
turtle.width(6)#The wirdth of the line draw is '6' - quite a thick line
turtle.up()#Here I start to position the turtle for the drawing of the pumpkin
turtle.goto(0,-200)
Let's begin the animation...
#Draw the pumpkin
#Draw right half of pumpkin
turtle.down()
turtle.right(20)
turtle.begin_fill()
turtle.circle(150,220)
#Draw left half of pumpkin
turtle.right(40)
turtle.circle(150,220)
turtle.end_fill()
#Draw lines on pumpkin
turtle.left(20)
turtle.circle(190,95)
turtle.up()
turtle.left(35)
turtle.forward(40)
turtle.down()
turtle.left(45)
turtle.circle(180,110)
#Reposition the turtle to draw the stalk
turtle.up()
turtle.goto(0,120)
turtle.right(45)
#Set up turtle to draw stalk
turtle.color("#004200")
turtle.fillcolor("darkgreen")
turtle.width(30)
#Draw stalk
turtle.down()
turtle.forward(40)
turtle.right(180)
turtle.width(20)
turtle.color("darkgreen")
turtle.forward(38)
turtle.right(215)
turtle.color("#004200")
turtle.width(25)
turtle.forward(50)
turtle.right(180)
turtle.width(15)
turtle.color("darkgreen")
turtle.forward(45)
turtle.right(215)
turtle.color("#004200")
turtle.width(20)
turtle.forward(40)
turtle.right(180)
turtle.width(10)
turtle.color("darkgreen")
turtle.forward(35)
turtle.left(300)
turtle.color("#004200")
turtle.width(20)
turtle.forward(40)
turtle.right(180)
turtle.width(10)
turtle.color("darkgreen")
turtle.forward(35)
#Reposition the turtle to draw the cutouts
turtle.up()
turtle.goto(50,0)
turtle.right(150)
#Set up turtle to draw eyes
turtle.color("#B74900")
turtle.fillcolor("#370037")
turtle.width(6)
turtle.down()
#Draw right eye
turtle.begin_fill()
while count<3:
turtle.forward(90)
turtle.right(120)
count=count+1
else:
count=0
turtle.end_fill()
#Reposition the turtle
turtle.up()
turtle.goto(-140,0)
#Draw left eye
turtle.down()
turtle.begin_fill()
while count<3:
turtle.forward(90)
turtle.right(120)
count=count+1
else:
count=0
turtle.end_fill()
#Reposition the turtle
turtle.up()
turtle.goto(-35,-100)
turtle.right(180)
#Draw nose
turtle.down()
turtle.begin_fill()
while count<3:
turtle.right(120)
turtle.forward(70)
count=count+1
else:
count=0
turtle.end_fill()
#Reposition the turtle
turtle.up()
turtle.goto(-130,-140)
turtle.right(180)
#Draw mouth
turtle.down()
turtle.begin_fill()
while count<5:
turtle.right(60)
turtle.forward(50)
turtle.left(120)
turtle.forward(50)
turtle.right(60)
count=count+1
else:
count=0
turtle.right(180)
turtle.forward(250)
turtle.end_fill()

... and here is the final result!