PYTHON: A Turtle Graphics Project
A Halloween Greeting 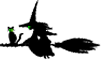
Pentacles in the sky (October 2022)
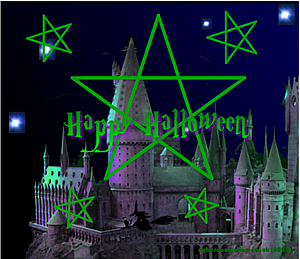
We start off by importing the turtle module into our program:
import turtle
We now have to set up the screen.
I have a graphic background for this program. I have also set the cursor to be a witch on a bloom (with her cat).
Both of these images have to be imported from my Trinket library - it takes png and gif images.
You can see how I incorporate the graphics below:
screen=turtle.Screen()
screen.setup(550,450)
# set the screen background
screen.bgpic("hogwarts2.png")
Now I add a graphic to identify the program as having been written by me
screen.addshape("logo.png")#add the logo for the base of the picture
logo=turtle.Turtle("logo.png")#The 'logo' Turtle
logo.shape("logo.png")
logo.up()#It is necassary to lift up the turtle to move it without making a line on the screen
logo.rt(270)
logo.goto(150,-210)
logo.down()#Now it is where I want it - I can put it down!
We need to set the cursor to be a witch on a broom and decide on its colour and the thickness of the line it draws - width()
screen.addshape("witchOnBroom60right2.png")
witch = turtle.Turtle("witchOnBroom60right2.png")
witch.shape("witchOnBroom60right2.png")
witch.color("#00CC66")
witch.width(5)
It is a good idea to set variables that you are going to use to zero before you start. Each time you use it remember to reset it to zero.
count=0
Now to make the witch draw the figure...
First we need to move her to the place we want to start from.
Initially she is placed in the centre of the screen.
When drawing a pentacle you start at the top - so the witch needs moving up the screen.... the pen needs to be 'up' for this or moving her will draw a line. So I turn her round and round then take her to where I want her to start from.
witch.up()
witch.lt(450)
witch.forward(200)
I then put the pen down and make sure she faces the correct direction to begin drawing.
witch.down()
witch.lt(162)
A pentacle is made of five lines that are equal in length and require a 'turn' of the same angle each time - so, it makes sense to use a 'while/else' command.
We need to count the number of times we repeat the process, so we use the variable 'count' - resetting it to zero as soon as we have completed the five strokes of the computer 'pen'.
while count<5:
witch.forward(300)
witch.rt(216)
count=count+1
else:count=0
After getting the first pentacle to 'work' I decided to draw a few more at different locations on the screen - different sizes too - as they are smaller, the line needs to be thinner...
witch.width(3)#smaller pentacles will be drawn with a thinner line
In between each I needed to lift the pen and reloacte the witch before putting the pen down again.
witch.up()
witch.rt(432)
witch.forward(200)
witch.down()
witch.lt(90)
while count<5:
witch.forward(80)
witch.rt(216)
count=count+1
else:count=0
A further pentacle...
witch.up()
witch.rt(682)
witch.forward(400)
witch.down()
while count<5:
witch.forward(60)
witch.rt(216)
count=count+1
else:count=0
... and another...
witch.up()
witch.rt(132)
witch.forward(200)
witch.down()
witch.lt(90)
while count<5:
witch.forward(70)
witch.rt(216)
count=count+1
else:count=0
... and another!
witch.up()
witch.rt(230)
witch.forward(400)
witch.down()
witch.lt(90)
while count<5:
witch.forward(90)
witch.rt(216)
count=count+1
else:count=0
Finally, I make the witch move to a suitable position on the background graphic - and call on a text graphic to wish you a Happy Halloween.
To do this I have to set it as another turtle.
witch.up()
witch.lt(110)
witch.forward(340)
witch.rt(45)
screen.addshape("HappyHalloween.png") #Here I am defining the greaphic and calling it onto the screen
text = turtle.Turtle("HappyHalloween.png")
text.shape("HappyHalloween.png")
text.rt(630)

And here we have the final result! Playing with turtles is such fun! Enjoy!